Selenium WebDriver Cheat Sheet Cheat Sheet. Selenium WebDriver Code Examples in Java Programming (Helpful to refer this sheet before attending Interviews) https.
- Selenium WebDriver Commands Cheat sheet. June 14, 2020 Following is the complete list of all commands available in Selenium WebDriver with their usage.
- All cheat sheets, round-ups, quick reference cards, quick reference guides and quick reference sheets in one page. XPath, CSS, DOM and Selenium: The Rosetta Stone.
Python selenium commands cheat sheet
Frequently used python selenium commands – Cheat Sheet
To import webdriver module in python use below import statement
Driver setup:
Firefox:
firefoxdriver = webdriver.Firefox(executable_path=”Path to Firefox driver”)
To download: Visit GitHub
Chrome:
chromedriver = webdriver.Chrome(executable_path=”Path to Chrome driver”)
To download: Visit Here
Internet Explorer:
iedriver = webdriver.IE(executable_path=”Path To IEDriverServer.exe”)
To download: Visit Here
Edge:
edgedriver = webdriver.Edge(executable_path=”Path To MicrosoftWebDriver.exe”)
To download: Visit Here
Opera:
operadriver = webdriver.Opera(executable_path=”Path To operadriver”)
To download: visit GitHub
Safari:
SafariDriver now requires manual installation of the extension prior to automation
Browser Arguments:
–headless
To open browser in headless mode. Works in both Chrome and Firefox browser
–start-maximized
To start browser maximized to screen. Requires only for Chrome browser. Firefox by default starts maximized
–incognito
To open private chrome browser
–disable-notifications
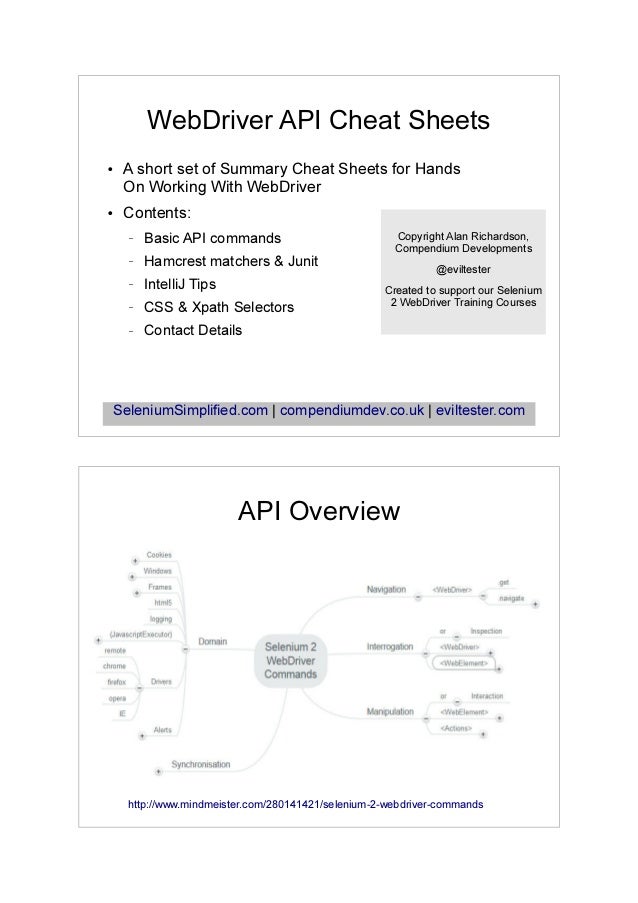
To disable notifications, works Only in Chrome browser
Example:
or
To Auto Download in Chrome:
To Auto Download in Firefox:
We can add any MIME types in the list. MIME for few types of files are given below.
- Text File (.txt) – text/plain
- PDF File (.pdf) – application/pdf
- CSV File (.csv) – text/csv or “application/csv”
- MS Excel File (.xlsx) – application/vnd.openxmlformats-officedocument.spreadsheetml.sheet or application/vnd.ms-excel
- MS word File (.docx) – application/vnd.openxmlformats-officedocument.wordprocessingml.document
Zip file (.zip) – application/zip
Note:
The value of browser.download.folderList can be set to either 0, 1, or 2.
0 – Files will be downloaded on the user’s desktop.
1 – Files will be downloaded in the Downloads folder.
2 – Files will be stored on the location specified for the most recent download
Disable notifications in Firefox
firefoxOptions.set_preference(“dom.webnotifications.serviceworker.enabled”, false);
firefoxOptions.set_preference(“dom.webnotifications.enabled”, false);
Open specific Firefox browser using Binary:
Open specific Chrome browser using Binary:
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
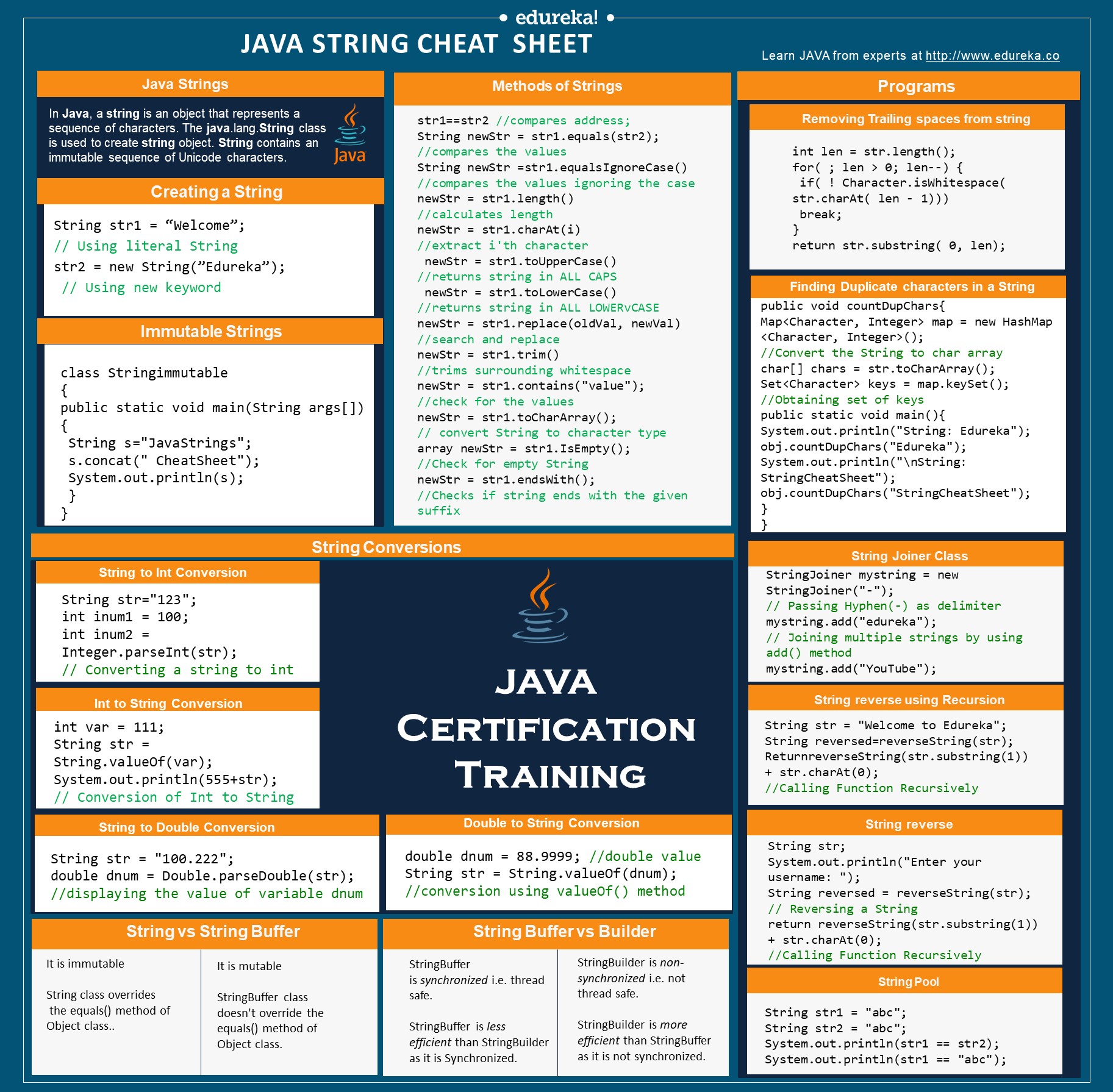
options = Options()
options.binary_location = “”
driver = webdriver.Chrome(chrome_options=options, executable_path=””)
driver.get(‘http://google.com/’)
Read Browser Details:
driver.title
driver.window_handles
driver.current_window_handles
driver.current_url
driver.page_source
Go to a specified URL:
driver.get(“http://google.com”)
driver.back()
driver.forward()
driver.refresh()
Locating Elements:
driver.find_element_by_ – To find the first element matching the given locator argument. Returns a WebElement
driver.find_elements_by_ – To find all elements matching the given locator argument. Returns a list of WebElement
By ID
<input id=”q” type=”text” />
element = driver.find_element_by_id(“q”)
By Name
<input id=”q” name=”search” type=”text” />
element = driver.find_element_by_name(“search”)
By Class Name
<div class=”username” style=”display: block;”>…</div>
element = driver.find_element_by_class_name(“username”)
By Tag Name
<div class=”username” style=”display: block;”>…</div>
element = driver.find_element_by_tag_name(“div”)
By Link Text
<a href=”#”>Refresh</a>
element = driver.find_element_by_link_text(“Refresh”)
By Partial Link Text
<a href=”#”>Refresh Here</a>
element = driver.find_element_by_partial_link_text(“Refresh”)
By XPath
<form id=”testform” action=”submit” method=”get”>
Username: <input type=”text” />
Password: <input type=”password” />
</form>
element = driver.find_element_by_xpath(“//form[@id=’testform’]/input[1]”)
By CSS Selector
<form id=”testform” action=”submit” method=”get”>
<input class=”username” type=”text” />
<input class=”password” type=”password” />
</form>
element = driver.find_element_by_css_selector(“form#testform>input.username”)
Important Modules to Import:
from selenium import webdriver
from selenium.webdriver.support.wait import WebDriverWait
from selenium.webdriver.support import expected_conditions
from selenium.webdriver.support.ui import Select

from selenium.webdriver.common.by import By
from selenium.webdriver.common.action_chains import ActionChains
from selenium.common.exceptions import NoSuchElementException
from selenium.webdriver.firefox.firefox_binary import FirefoxBinary
from selenium.webdriver.chrome.options import Options
from selenium.webdriver.firefox.options import Options
Python Selenium commands for operation on elements:
button/link/image:
click()
get_attribute()
is_displayed()
is_enabled()
Text field:
send_keys()
clear()
Checkbox/Radio:
is_selected()
click()
Select:
Find out the select element using any element locating strategies and then select options from list using index, visible text or option value.
Element properties:
is_displayed()
is_selected()
is_enabled()
These methods return either true or false.
Read Attribute:
get_attribute(“”)
Get attribute from a disabled text box
driver.find_element_by_id(“id”).get_attribute(“value”);
Screenshot:
Note: An important note to store screenshots is that save_screenshot(‘filename’) and get_screenshot_as_file(‘filename’) will work only when extension of file is ‘.png’. Otherwise content of the screenshot can’t be viewed
Read articles for more details about taking screenshot and element screenshot
The list here contains mostly used python selenium commands but not exhaustive. Please feel free to add in comments if you feel something is missing and should be here.
3 Responses
[…] Previous: Previous post: Execute Python Selenium tests in Selenium GridNext: Next post: Python selenium commands cheat sheet […]
Thank you very much
Hi Sir,
I am trying to do the sorting in selenium with python using For loop could u please help me is there any way that i can do it ?
Sorting in descending order in the below website
website: https://jqueryui.com/sortable/
For Python version the link is here
Install Java
To install Java go to this link
IDE
You can use any text editor. I recommend Eclipse as it is free and have extensive support. For list of popular editors , this are the links
Download Selenium
Download selenium webdriver in this link
Dirty our hands !
Import Selenium
Browsers support (Firefox , Chrome , Internet Explorer, Edge , Opera)
Driver setup:
Chrome:
System.setProperty('webdriver.chrome.driver', “'Path To chromedriver');
To download: Visit Here
Firefox:
System.setProperty('webdriver.gecko.driver', 'Path To geckodriver');
To download: Visit GitHub
Internet Explorer:
System.setProperty('webdriver.ie.driver', 'Path To IEDriverServer.exe');
To download: Visit Here
Edge:
System.setProperty('webdriver.edge.driver', 'Path To MicrosoftWebDriver.exe');
To download: Visit Here
Opera:
System.setProperty('webdriver.opera.driver', 'Path To operadriver');
To download: visit GitHub
Browser Arguments:
–headless
To open browser in headless mode. Works in both Chrome and Firefox browser
–start-maximized
To start browser maximized to screen. Requires only for Chrome browser. Firefox by default starts maximized
–incognito
To open private chrome browser
–disable-notifications
To disable notifications, works Only in Chrome browser
Example:
Alternative
Launch URL
Retrieve Browser Details:
Navigation
Locating Elements
By id
<input id=”login” type=”text” />
By Class Name
<input class=”gLFyf” type=”text” />
By Name
<input name=”z” type=”text” />
By Tag Name
<div id=”login” >…</div>
By Link Text
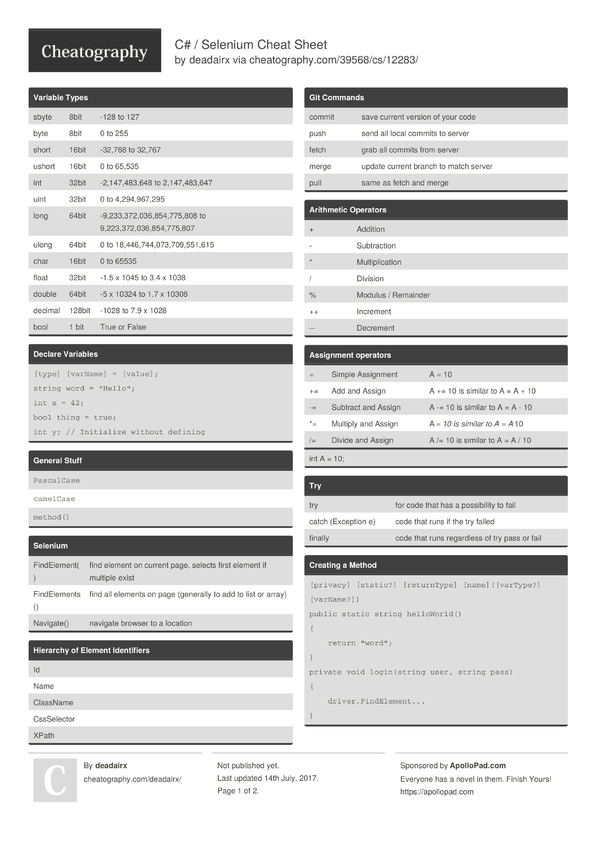
<a href=”#”>News</a>
By XPath
<form id=”login” action=”submit” method=”get”>
Username: <input type=”text” />
Password: <input type=”password” />
</form>
By CSS Selector
<form id=”login” action=”submit” method=”get”>
Username: <input type=”text” />
Password: <input type=”password” />
</form>
Clicking / Input text
Clicking button
Send Text
Waits
Implicit Waits
An implicit wait instructs Selenium WebDriver to poll DOM for a certain amount of time, this time can be specified, when trying to find an element or elements that are not available immediately.
Explicit Waits
Explicit wait make the webdriver wait until certain conditions are fulfilled . Example of a wait
List of explicit waits
- alertIsPresent()
- elementSelectionStateToBe()
- elementToBeClickable()
- elementToBeSelected()
- frameToBeAvaliableAndSwitchToIt()
- invisibilityOfTheElementLocated()
- invisibilityOfElementWithText()
- presenceOfAllElementsLocatedBy()
- presenceOfElementLocated()
- textToBePresentInElement()
- textToBePresentInElementLocated()
- textToBePresentInElementValue()
- titleIs()
- titleContains()
- visibilityOf()
- visibilityOfAllElements()
- visibilityOfAllElementsLocatedBy()
- visibilityOfElementLocated()
Selenium Cheat Sheet Python
Loading a list of elements like li and selecting one of the element

Read Attribute
Get CSS
CSS values varies on different browser, you may not get same values for all the browser.
Capture Screenshot
This will saved the file as in the path of destFile.
isSelected()
isSelected() method in selenium verifies if an element (such as checkbox) is selected or not. isSelected() method returns a boolean.
Selenium Commands Pdf
isDisplayed()
isDisplayed() method in selenium webdriver verifies and returns a boolean based on the state of the element (such as button) whether it is displayed or not.
isEnabled()
is_enabled() method in selenium python verifies and returns a boolean based on the state of the element (such as button) whether it is enabled or not.
Selenium Cheat Sheet Python
Minimum modules to import
Created : 17 December 2019
